SwiftUI coding conventions: On closure blocks
It is the tabs vs. spaces of SwiftUI and an excellent new opportunity to do a little ↗ bike-shedding:
How to format closure blocks when writing SwiftUI code?
Let's look at some example code from Apple taken from ↗ sheet(isPresented:onDismiss:content:). I am a bit opinionated about this and here is how I recommend to do it.
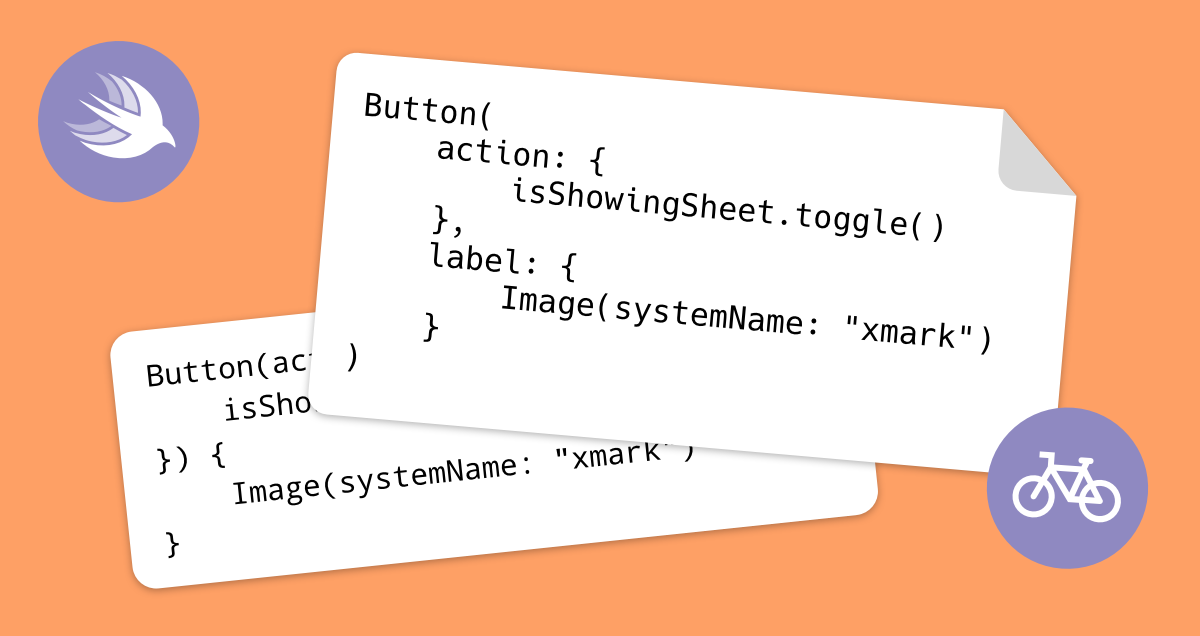
1. When to use trailing closures
Example code:
Button("Dismiss",
action: { isShowingSheet.toggle() })
In cases where all the non-closure arguments can fit on one line and the meaning of the closure is self-explanatory, I use a trailing closure:
Button("Dismiss") {
isShowingSheet.toggle()
}
2. When not to use trailing closures
Example code:
Button(action: {
isShowingSheet.toggle()
}) {
Image(systemName: "info.circle.fill")
}
.sheet(isPresented: $isShowingSheet,
onDismiss: didDismiss) {
VStack {
Text("License Agreement")
.font(.title)
.padding(50)
Text("""
Terms and conditions go here.
""")
.padding(50)
Button("Dismiss",
action: { isShowingSheet.toggle() })
}
}
If there are multiple closure arguments, or when it's more readable to write every argument on its own line, or if the meaning of the closure is not self-explanatory, I don't use a trailing closure:
Button(
action: {
isShowingSheet.toggle()
},
label: {
Image(systemName: "info.circle.fill")
}
)
.sheet(
isPresented: $isShowingSheet,
onDismiss: didDismiss,
content: {
VStack {
Text("License Agreement")
.font(.title)
.padding(50)
Text(
"""
Terms and conditions go here.
"""
)
.padding(50)
Button("Dismiss") {
isShowingSheet.toggle()
}
}
}
)