Adding external libraries to iOS projects with the Swift Package Manager
The Swift Package Manager can be used to add external libraries in Xcode projects. This makes the tool a good alternative to Cocoapods and Carthage for managing the dependencies of an iOS project.
The following tutorial shows how to use the Swift Package Manager by adding the 3rd party library Kingfisher for asynchronous image loading to an example project. This library provides the metadata required for the Swift Package Manager, recognizable by the file Package.swift in the root folder of the project.
-
Download the starter project for the sample project Countries. This contains the basic structure for an app that displays images using the SwiftUI List view:
-
Open the Kingfisher project page and copy the clone URL of the project:
-
In Xcode use File » Swift Packages » Add Package Dependency… to add a new dependency:
-
Specify the clone URL of the Kingfisher library:
-
Leave the default version settings - the library will be updated until the next major release:
-
Add the Kingfisher target to the app target:
-
-
Have a look at Swift Packages in the project settings. Here, the package configuration can be changed later:
-
Use the library in the CountryRowView: Import the Kingfisher module there and use the KFImage class to show the images downloaded from the URL from the data model:
import SwiftUI import Kingfisher struct CountryRowView: View { let country: Country var body: some View { HStack { KFImage(country.landmark.imageUrl) .resizable() .scaledToFit() .frame(width: 50) Text(self.country.name) Spacer() } } }
Q & A
Where are the checked out modules stored?
In the Derived Data folder of Xcode:

What happens if the project is opened without the library having been downloaded?
The library will be automatically downloaded by Xcode:
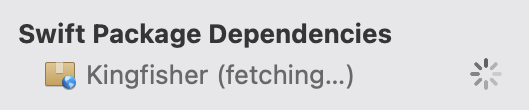
What happens when a new version of the library is released?
The commit hash of the library is stored in the Xcode project under project.xcworkspace/xcshareddata/swiftpm/Package.resolved. This declaration is used to download the library. There is no automatic update, the version of the library will be fixed.
The dependency can be updated with File » Swift Packages » Update to Latest Package Versions.
More information
-
Swift Package ManagerDocumentation for the Swift Package Manager.
-
Swift Package Manager: UsageDocumentation on how to use the Swift Package Manager in the SPM GitHub project.
-
WWDC 2019: Adopting Swift Packages in XcodePresentation from WWDC 2019 on the Swift Package Manager.
-
WWDC 2019: Creating Swift PackagesPresentation from WWDC 2019 on the creation of Swift Packages.
-
AsyncImageHint: In iOS 15, SwiftUI can load images asynchronously from a URL with the AsyncImage view. So using the Kingfisher library only makes sense in projects that need to be compatible with older iOS versions.